How To Add Custom Fonts to Your Next.js and Tailwind CSS Project
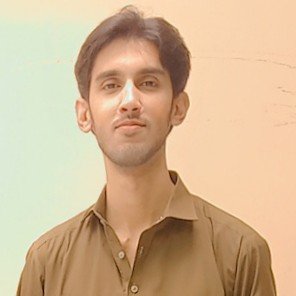
Waleed Mumtaz
@waleedmumtaazPublished on
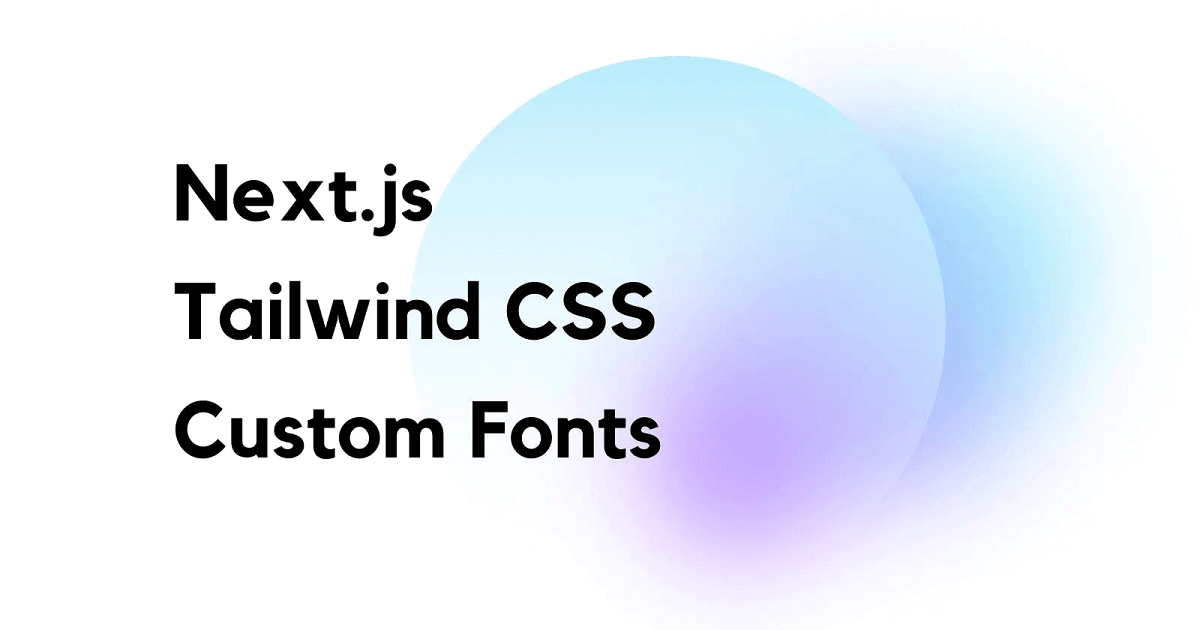
A unique-looking font on a webpage really makes it stand out. You might even consider using a custom font for your website as it makes it visually distinct and becomes a part of the identity of your brand.
Table of Contents
- Install Next.js
- Install Tailwind CSS
- Configure Tailwind CSS
- Add Your Custom Font Files
- Add Font In Your CSS
- Extend Tailwind’s theme Object to Add Font
- Time to Use Our Font!
I recently implemented a custom font on a Next.js website using Tailwind CSS. I thought of it as the perfect opportunity to write about it and write my first blog post. 😀
Here it goes!
Note: If you already have Next.js installed and configured with Tailwind CSS, you might want to skip to the Add Your Custom Font Files section below.
Install Next.js
We begin by installing Next.js using the terminal. Create your Next.js App with the following command. Feel free to replace my-next-project
with any other project name of your choice.
npx create-next-app my-next-project
cd into your project directory
cd my-next-project
We then open our project in VS Code with the following command.
code .
You can also open your project in any other Editor of your choice if you do not use VS Code.
Install Tailwind CSS
After the project is open in VS Code, we use VS Code’s Integrated Terminal to install Tailwind CSS.
npm install -D tailwindcss postcss autoprefixer
This will install the latest version of Tailwind CSS (v3.0.7 as of writing this article), along with its peer dependencies, postcss
and autoprefixer
.
Configure Tailwind CSS
Generate tailwind.config.js
and postcss.config.js
files with the following command.
npx tailwindcss init -p
Next, open your tailwind.config.js
file that was just generated. This is how your file will look like:
module.exports = {
content: [],
theme: {
extend: {}
},
plugins: []
};
Add the following paths in the content
array
module.exports = {
content: ['./pages/**/*.{js,ts,jsx,tsx}', './components/**/*.{js,ts,jsx,tsx}'],
theme: {
extend: {}
},
plugins: []
};
Note: As of Tailwind CSS v3.0, purge
has been replaced by content
. You can add the same paths in your purge
array if you are using an earlier version of Tailwind CSS.
Replace the contents of globals.css
with the following Tailwind directives:
@tailwind base;
@tailwind components;
@tailwind utilities;
Also, make sure that globals.css
is being imported into your _app.js
file.
Add Your Custom Font Files
For this example, I will be using the Snow Blue font by Girinesia.
Snow Blue is an OpenType font. You can read more about other font MIME types here at MDN Web Docs.
If you already have a custom font file, add it under public/fonts
directory in your project.
Add Font In Your CSS
We now add our custom font in our globals.css
file by using Tailwind CSS’ @layer base
directive. Make sure to add this under the other three Tailwind directives already present in the file.
@tailwind base;
@tailwind components;
@tailwind utilities;
@layer base {
@font-face {
font-family: 'Snow Blue';
src: url('/fonts/SnowBlue.otf') format('opentype');
}
}
Extend Tailwind’s theme
Object to Add Font
Finally, we extend Tailwind’s theme
object to add our custom font so that we can use it in our project.
In the tailwind.config.js
file, add the following fontFamily
object inside the theme
object by extending it.
module.exports = {
content: ['./pages/**/*.{js,ts,jsx,tsx}', './components/**/*.{js,ts,jsx,tsx}'],
theme: {
extend: {
fontFamily: {
'snow-blue': ['Snow Blue', 'sans-serif']
}
}
},
plugins: []
};
Time to Use Our Font!
Make sure to run your dev server:
npm run dev
And open your browser and go to localhost:3000
Here is how I have my index.js
file structured.
export default function Home() {
return (
<>
<div className="grid h-screen w-screen place-items-center">
<h1 className="text-9xl text-sky-500">Winter Is Here 🥶</h1>
</div>
</>
);
}
The output:
Now we use our snow-blue
font:
export default function Home() {
return (
<>
<div className="grid h-screen w-screen place-items-center">
<h1 className="font-snow-blue text-9xl text-sky-500">Winter Is Here 🥶</h1>
</div>
</>
);
}
The output:
That’s it! As simple as that. 😎